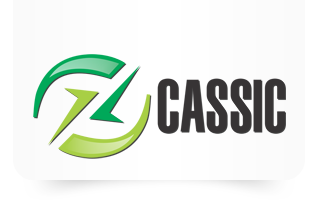
Segundo Componente Visual
Este componente será usado para receber e validar CNPJ e CPF. Assim como o TMaskEdit nosso componente terá uma mascara de edição.
Novamente repita todos os procedimentos do primeiro componente criado mudando apenas as opções abaixo:
Ancestor Component: TCustomMaskEdit
Class Name: TCNPJCPFEdit
Pallete Page: CursoDelphi
Unit File Name: CNPJCPFEdit.pas
Inclua o código abaixo:
01 |
unit CNPJCPFEdit; |
02 |
|
03 |
interface |
04 |
|
05 |
uses |
06 |
SysUtils, Classes, Controls, StdCtrls, Mask, Dialogs; |
07 |
|
08 |
type |
09 |
TTipoDoc = (tdCNPJ, tdCPF); |
10 |
|
11 |
TCNPJCPFEdit = class(TCustomMaskEdit) |
12 |
private |
13 |
{ Private declarations } |
14 |
procedure cmExit(var message: TCMExit); message cm_Exit; |
15 |
procedure ConfigEditMask; |
16 |
function CNPJ: Boolean; |
17 |
function CPF: Boolean; |
18 |
protected |
19 |
{ Protected declarations } |
20 |
public |
21 |
{ Public declarations } |
22 |
constructor Create(AOwner: TComponent); override; |
23 |
published |
24 |
{ Published declarations } |
25 |
property TipoDoc: TTipoDoc; |
26 |
property WidthCNPJ: Integer; |
27 |
property WidthCPF: Integer; |
28 |
property OnChange; |
29 |
property OnClick; |
30 |
property OnDblClick; |
31 |
property OnDragDrop; |
32 |
property OnDragOver; |
33 |
property OnEndDock; |
34 |
property OnEndDrag; |
35 |
property OnEnter; |
36 |
property OnExit; |
37 |
property OnKeyDown; |
38 |
property OnKeyPress; |
39 |
property OnKeyUp; |
40 |
property OnMouseDown; |
41 |
property OnMouseMove; |
42 |
property OnMouseUp; |
43 |
property OnStartDock; |
44 |
property OnStartDrag; |
45 |
end; |
46 |
|
47 |
procedure Register; |
48 |
|
49 |
implementation |
50 |
|
51 |
procedure Register; |
52 |
begin |
53 |
RegisterComponents('CursoDelphi', [TCNPJCPFEdit]); |
54 |
end; |
55 |
|
56 |
end. |
Pressione Ctrl+Shift+C e continue incluindo o código mostrado:
001 |
unit CNPJCPFEdit; |
002 |
|
003 |
interface |
004 |
|
005 |
uses |
006 |
SysUtils, Classes, Controls, StdCtrls, Mask, Dialogs; |
007 |
|
008 |
type |
009 |
TTipoDoc = (tdCNPJ, tdCPF); |
010 |
|
011 |
TCNPJCPFEdit = class(TCustomMaskEdit) |
012 |
private |
013 |
FWidthCNPJ: Integer; |
014 |
FWidthCPF: Integer; |
015 |
FTipoDoc: TipoDoc; |
016 |
procedure SetTipoDoc(const Value: TTipoDoc); |
017 |
procedure SetWidthCNPJ(const Value: Integer); |
018 |
procedure SetWidthCPF(const Value: Integer); |
019 |
{ Private declarations } |
020 |
procedure cmExit(var message: TCMExit); message cm_Exit; |
021 |
procedure ConfigEditMask; |
022 |
function CNPJ: Boolean; |
023 |
function CPF: Boolean; |
024 |
protected |
025 |
{ Protected declarations } |
026 |
public |
027 |
{ Public declarations } |
028 |
constructor Create(AOwner: TComponent); override; |
029 |
published |
030 |
{ Published declarations } |
031 |
property TipoDoc: TTipoDoc read FTipoDoc write SetTipoDoc; |
032 |
property WidthCNPJ: Integer read FWidthCNPJ write SetWidthCNPJ; |
033 |
property WidthCPF: Integer read FWidthCPF write SetWidthCPF; |
034 |
property OnChange; |
035 |
property OnClick; |
036 |
property OnDblClick; |
037 |
property OnDragDrop; |
038 |
property OnDragOver; |
039 |
property OnEndDock; |
040 |
property OnEndDrag; |
041 |
property OnEnter; |
042 |
property OnExit; |
043 |
property OnKeyDown; |
044 |
property OnKeyPress; |
045 |
property OnKeyUp; |
046 |
property OnMouseDown; |
047 |
property OnMouseMove; |
048 |
property OnMouseUp; |
049 |
property OnStartDock; |
050 |
property OnStartDrag; |
051 |
end; |
052 |
|
053 |
procedure Register; |
054 |
|
055 |
implementation |
056 |
|
057 |
procedure Register; |
058 |
begin |
059 |
RegisterComponents('CursoDelphi', [TCNPJCPFEdit]); |
060 |
end; |
061 |
|
062 |
{ TCNPJCPFEdit } |
063 |
|
064 |
procedure TCNPJCPFEdit.cmExit(var message: TCMExit); |
065 |
begin |
066 |
case Self.FTipoDoc of |
067 |
tdCNPJ:if not( CNPJ ) then Self.SetFocus; |
068 |
tdCPF:if not( CPF ) then Self.SetFocus; |
069 |
end; |
070 |
end; |
071 |
|
072 |
function TCNPJCPFEdit.CNPJ: Boolean; |
073 |
var |
074 |
n1, n2, n3, n4, n5, n6, n7, n8, n9, n10, n11, n12, d1, d2: Integer; |
075 |
Num, Digitado, Calculado: string; |
076 |
begin |
077 |
Num := StringReplace(Self.Text, '.', '', [rfReplaceAll]); |
078 |
Num := StringReplace(Num, '-', '', [rfReplaceAll]); |
079 |
Num := StringReplace(Num, '/', '', [rfReplaceAll]); |
080 |
if ( Trim( Num ) = '' ) then |
081 |
begin |
082 |
Result := True; |
083 |
Exit; |
084 |
end; |
085 |
if ( Length( Num ) <> 14 ) then |
086 |
begin |
087 |
MessageDlg('CNPJ incompleto. Digite novamente.', |
088 |
mtError, [mbOk], 0 ); |
089 |
Result := False; |
090 |
Exit; |
091 |
end; |
092 |
n1 := StrToInt( Num[1] ); |
093 |
n2 := StrToInt( Num[2] ); |
094 |
n3 := StrToInt( Num[3] ); |
095 |
n4 := StrToInt( Num[4] ); |
096 |
n5 := StrToInt( Num[5] ); |
097 |
n6 := StrToInt( Num[6] ); |
098 |
n7 := StrToInt( Num[7] ); |
099 |
n8 := StrToInt( Num[8] ); |
100 |
n9 := StrToInt( Num[9] ); |
101 |
n10 := StrToInt( Num[10] ); |
102 |
n11 := StrToInt( Num[11] ); |
103 |
n12 := StrToInt( Num[12] ); |
104 |
d1 := n12*2+n11*3+n10*4+n9*5+n8*6+ |
105 |
n7*7+n6*8+n5*9+n4*2+n3*3+n2*4+n1*5; |
106 |
d1 := 11 - ( d1 mod 11 ); |
107 |
if ( d1 >= 10 ) then d1 := 0; |
108 |
d2 := d1*2+n12*3+n11*4+n10*5+n9*6+n8*7+ |
109 |
n7*8+n6*9+n5*2+n4*3+n3*4+n2*5+n1*6; |
110 |
d2 := 11 - ( d2 mod 11 ); |
111 |
if ( d2 >= 10 ) then d2 := 0; |
112 |
Calculado := IntToStr(d1) + IntToStr(d2); |
113 |
Digitado := Num[13] + Num[14]; |
114 |
if ( Calculado = Digitado ) then Result := True |
115 |
else |
116 |
begin |
117 |
MessageDlg('CNPJ inválido.', mtError, [mbOk], 0); |
118 |
Result := False; |
119 |
end; |
120 |
end; |
121 |
|
122 |
procedure TCNPJCPFEdit.ConfigEditMask; |
123 |
begin |
124 |
Self.Clear; |
125 |
case FTipoDoc of |
126 |
tdCNPJ: |
127 |
begin |
128 |
Self.EditMask := '!99.999.999/9999-99;1;_'; |
129 |
Self.Width := WidthCNPJ; |
130 |
end; |
131 |
tdCPF: |
132 |
begin |
133 |
Self.EditMask := '!999.999.999-99;1;_'; |
134 |
Self.Width := WidthCPF; |
135 |
end; |
136 |
end; |
137 |
end; |
138 |
|
139 |
function TCNPJCPFEdit.CPF: Boolean; |
140 |
var |
141 |
n1, n2, n3, n4, n5, n6, n7, n8, n9, d1, d2: Integer; |
142 |
Num, Digitado, Calculado: string; |
143 |
begin |
144 |
Num := StringReplace(Self.Text, '.', '', [rfReplaceAll]); |
145 |
Num := StringReplace(Num, '-', '', [rfReplaceAll]); |
146 |
if ( Trim( Num ) = '' ) then |
147 |
begin |
148 |
Result := True; |
149 |
Exit; |
150 |
end; |
151 |
if ( Length( Num ) <> 11 ) then |
152 |
begin |
153 |
MessageDlg('CPF incompleto. Digite novamente.', |
154 |
mtError, [mbOk], 0); |
155 |
Result := False; |
156 |
Exit; |
157 |
end; |
158 |
n1 := StrToInt( Num[1] ); |
159 |
n2 := StrToInt( Num[2] ); |
160 |
n3 := StrToInt( Num[3] ); |
161 |
n4 := StrToInt( Num[4] ); |
162 |
n5 := StrToInt( Num[5] ); |
163 |
n6 := StrToInt( Num[6] ); |
164 |
n7 := StrToInt( Num[7] ); |
165 |
n8 := StrToInt( Num[8] ); |
166 |
n9 := StrToInt( Num[9] ); |
167 |
d1 := n9*2+n8*3+n7*4+n6*5+n5*6+n4*7+n3*8+n2*9+n1*10; |
168 |
d1 := 11 - ( d1 mod 11 ); |
169 |
if ( d1 >= 10 ) then d1 := 0; |
170 |
d2 := d1*2+n9*3+n8*4+n7*5+n6*6+n5*7+n4*8+n3*9+n2*10+n1*11; |
171 |
d2 := 11 - ( d2 mod 11 ); |
172 |
if ( d2 >= 10 ) then d2 := 0; |
173 |
Calculado := IntToStr(d1) + IntToStr(d2); |
174 |
Digitado := Num[10] + Num[11]; |
175 |
if ( Calculado = Digitado ) then Result := True |
176 |
else |
177 |
begin |
178 |
MessageDlg('CPF inválido.', mtError, [mbOk], 0); |
179 |
Result := False; |
180 |
end; |
181 |
end; |
182 |
|
183 |
constructor TCNPJCPFEdit.Create(AOwner: TComponent); |
184 |
begin |
185 |
inherited; |
186 |
Self.FWidthCNPJ := 110; |
187 |
Self.FWidthCPF := 85; |
188 |
Self.FTipoDoc := tdCNPJ; |
189 |
Self.Width := WidthCNPJ; |
190 |
Self.Clear; |
191 |
Self.EditMask := '!99.999.999/9999-99;1;_'; |
192 |
end; |
193 |
|
194 |
procedure TCNPJCPFEdit.SetTipoDoc(const Value: TTipoDoc); |
195 |
begin |
196 |
FTipoDoc := Value; |
197 |
ConfigEditMask; |
198 |
end; |
199 |
|
200 |
procedure TCNPJCPFEdit.SetWidthCNPJ(const Value: Integer); |
201 |
begin |
202 |
FWidthCNPJ := Value; |
203 |
if ( Self.FTipoDoc = tdCNPJ ) then Width := Value; |
204 |
end; |
205 |
|
206 |
procedure TCNPJCPFEdit.SetWidthCPF(const Value: Integer); |
207 |
begin |
208 |
FWidthCPF := Value; |
209 |
if ( Self.FTipoDoc = tdCPF ) then Width := Value; |
210 |
end; |
211 |
|
212 |
end. |
Crie um arquivo DCR e um bitmap para o componente (Figura 1). Depois acrescente este componente ao nosso pacote dê um Build, não é precisando chamar o Install do pacote.
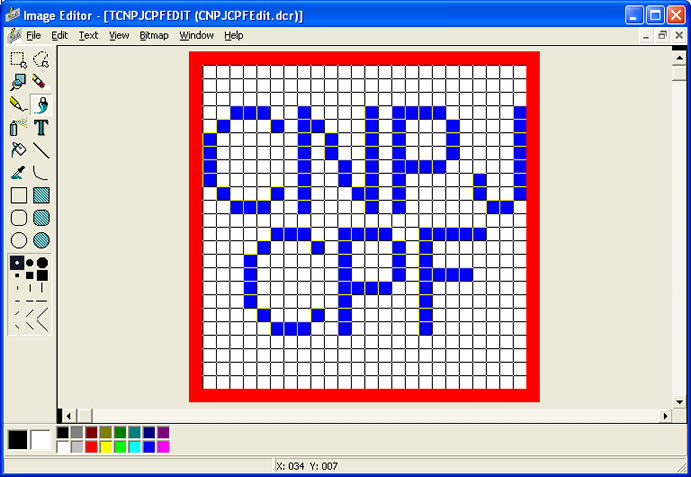
Figura 1 – Imagem do componente TCNPJCPFEdit